Adding Cells
Overview
- if CellValid and AreaDistortionOkay
- NumSidesOutside= number of consecutive adjacent cells that are OUTSIDE, UNKNOWN, or OFF_GRID
- If NumSidesOutside==1
- If there is another OUTSIDE, UNKNOWN, or OFF_GRID
opposite the one found
- Else
we have a NOTCH, PLATEAU, or GLEN
- If the new segments do not intersect with the existing bounds
- If NumSidesOutside=1 (Notch)
- If DistanceDistortionOkay
- If NumSidesOutside=2 (glen)
- If NewPoint is not in existing bounds
- If NumSidesOutside=3 (plateau)
- If new points are not in existing bounds
- If DistnceDistortionOkay
- If Segments do not intersect with each other
- New bounds points are not alredy in the current bounds
- Insert 2 new bounds points
-
Data Structures
There is a series of 2d arrays that contains information on the cells, corner points, and distance distortions.
Variables
The NumColumns and NumRows is the number of entries in the Corner Point Arrays.
- NumColumns - number of columns in the Corner Point arrays
- NumRows - number of rows in the Corner Point arrays
Cell Arrays:
These arrays contain information each of the cells in the grid. The cell at 0,0 is in the lower-left of the grid (minimum latitude and longitude)
- Statuses[NumRows-1][NumColumns-1]
- AreaDistortions[NumRows-1][NumColumns-1]
- BoundsPoints[NumRows-1][NumColumns-1]: Contains references to the
Corner Point Arrays
These arrays contain data on the corner points that surround the cells. There is one additional column and row for the extra row and column of points on the top and right of the grid of points.
- Northings[NumRows][NumColumns]
- Eastings[NumRows][NumColumns]
- Latitudes[NumRows][NumColumns]
- Longitudes[NumRows][NumColumns]
Distance Distortion Arrays
The distortion arrays contain the distortion for the line segements connecting the corner points so there is one fewer column for the horizontal distances and one fewer row for the vertical distances.
- HorizontalDistanceDistortions[NumRow][NumColumns-1]
- VerticalDistanceDistortions-1[NumRow-1][NumColumns]
Offset Arrays
There are a set of arrays for accessing information when we add a cell. The cell offsets provide offsets to the cell indexes to find the cells that are adjacent to a cell of interest. The Point Offset arrays provide offstes for the point indexes to find the points that are at the corners of a cell.
The Cell Offset arrays provide indexes to find the adjacent cells starting with the one above the target cell and moving clockwise.
- ColumnCellOffsets=[ 0, 1, 0,-1, 0, 1, 0] # above, right, below, left
- RowCellOffsets= __[ 1, 0,-1, 0, 1, 0,-1]
Offsets to points in the cells around an existing cell. Note that the lower-left point is the same as the index to the cell
the upper right points is +1,+1 from the cell index
The Point Offset arrays provide indexes to the points that are at the corners of the cell. This starts in the top-right and moves clockwise. The Point Offset arrays index the points that are at the end of a segment that is common with an adjancet cell using the same index. Taking the Index-1 provides the segment at the start of the segment that is common.
- ColumnPointOffsets=[ 1, 1, 0, 0, 1, 1, 0, 0, 1, 1, 0, 0, 1, 1, 0, 0 ] # top-right, bottom-right, bottom-left, top-left, repeat
- RowPointOffsets=__[ 1, 0, 0, 1, 1, 0, 0, 1, 1, 0, 0, 1, 1, 0, 0, 1 ]
Adding the Origin Cells
Adding cells starts by adding the four cells that surround the origin. If these cells cannot be added, creating the desired bounds fails.
1. Finding the first inside cell
2. Adding the other 3 origin cells
Adding Additional Cells
The CellOffsets arrays are used to go "around" the target cell to find the first adjancet cell that is INSIDE the existing Desired Bounding Polygon. This sets:
Check Cell
1. Find the number of OUTSIDE cells that are on adjacent sides of the target cell
1.1 Find the first inside cell
1.2 Find the first outside cell after the first inside cell
Sets StartOutsidePointIndex
1.2 Finding the NumSidesOutside
Going from the Index, we continue around the target cell to find the last side that is shared with a cell that is INSIDE and the first side that is not INSIDE (i.e. OUTSIDE or UNKNOWN). This sets:
- NumSidesOutside: Number of cells that are outside and on adjacent sides
- NumSidesOutside - 0, 1, 2, or 3
- 0 happens when a cell is surrounded by outside cells. This can happen on the corner or sides of the grid.
- 1 happens if:
- there is a NOTCH in the inside cells or
- There are two inside cells and one is directly across from the other (really two outside cells but not on adjacent sides
- 2
- 3
- 4 could only happen on the first cell and is why _CheckCell() is not called on the first cell
2. Checks for all values of NumSidesOutside
2.1 If there is One Outside Cell (NOTCH or CANYON)
- If there is a cell oppositte the one we found
that is inside
- Don't add the cell
2.2 Check for intersections
If any of the sides that have an adjacent cell that is outside intersect the existing bounding polygon, we cannot make the cell INSIDE.
3. If there are two outside adjancet cells
3.1 One outside adjacent cell (NOTCH)
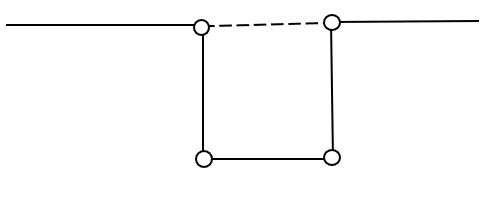
- Check for distance distortion
- Delete the two points on the inside of the NOTCH
Issue: Do we need to check for intersectinos with the bounds?
3.2 Two outside adjancent cells (GLEN)

For this case, there will be one new point that is added and one removed. This is implemented as moving the point.
- Do not move the point if:
- The new point is within the existing Desired Bounding Polygon
- The Distance Distortion is outside the desired limits
- The point is already in the bounds points (could happen when the projected space loops around on itself
-
4.3 If there are three outside adjacent cells (PLATEAU)
Do not add the points if:
- The new points are within the existing Desired Bounding Polygon
- The Distance Distortion is outside the desired limits
- The point is already in the bounds points (could happen when the projected space loops around on itself)
- The new segments intersect with each other
-