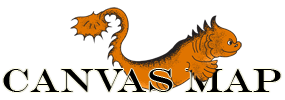
Customizing the Look of the Map
You can change everything about the look of a CanvasMap by changing element's styles, hidding elements, and replacing them. Below are a couple of simple topics to get you started.
Background Color of the Scene
The elements such as the map itself, use HTML 5 Canvas objects and you'll need to change some of these styles directly. This includes the background for the scene.
var TheScene=TheCanvasMap.GetScene(); TheScene.SetBackgroundColor("#8888ff");
Adding a Scale Bar
You can add a scale bar that is accurate for where it is place on the map. If you are using projected data, you'll need to make sure you specified a projector for the CanvasMap.
var TheScaleBar=new CMScaleBar(480,370,200,50); TheScene.AddMapElement(TheScaleBar);
The default units for the scale bar are SI units. You can change them to English units by calling:
TheScaleBar.SetUnits(CMScaleBar.UNITS_ENGLISH);
Change the Images used by CanvasMap
You can change the images including the tool icons, by changing the ones that are specified when you call SetImageFolder() or create a new folder with images that have the same names as those in the default folder that comes with CanvasMap.
Design
Overall Design
To go further, we need to look at how CanvasMap is designed. As you've already seen, CanvasMap relies upon an HTML page that includes:
- Includes for JavaScript files containing the CanvasMap code
- CSS file(s) for CanvasMap styleing
- "DIV" elements in the HTML for the CanvasMap(s)
The CSS file "CanvasMap.css" contains the style information for all of CanvasMap and the styles are named based on the names of the elements in CanvasMap (see below for a full list of the elements). The JavaScript files are the bulk of the CanvasMap code and are described below.
DOM Element Hierarchy
The list below shows how the elements within the CanvasMap are contained within each other. These definitions are used to access each of the elements with the GetElement() function. All of these elements can be customized, replaced, or hidden. Refer back to the first tutorial to see how each of these elements appears within a CanvasMap.
- MAP_CONTAINER
- contains all the elements in a CanvasMap
- MAP_HEADER - header across the top of the map.
- TOOL_CONTAINER
- contains the tools just below the header
- TOOLS_TITLE - "Toolbar" title
- TOOL_SELECT - arrow for selecting
- TOOL_PAN - hand for panning the map
- CANVAS_CONTAINER
- contains the HTML 5 Canvas element and map elements
- NAVIGATION - buttons to zoom in, zoom out, and go home
- CANVAS - the HTML 5 Canvas element that is used to paint the map
- TAB_CONTAINER - shows the tabs for "Layers", "Background", and "Search"
- LAYER_LIST - list of forground layers
- BACKGROUND_LIST - list of background layers
- SEARCH_PANEL - panel with the search entry and results
- MAP_FOOTER
- footer to show information at the bottom of the map
- SRS - element to display the spacial reference information
- COORDINATES - element to show the coordinates at the mouse cursor position
- MAP_CREDITS - text string that can include author and date
Not that the scale bar and north arrow do not appear in this list. This is because they need to be objects assocaited with the scene so they can use the projection engine to find their orientation and length.
Change the contents of an element
You can customize the contents of some of the elements, such as the MapHeader or ToolTitle. After calling Initialize() you can get the element and then set its HTML
1. Changing the Title
var MapHeader=TheCanvasMap.GetElement(CanvasMap.MAP_HEADER); // NOTE: This would seem to conflict with header title in canvas map. CanvasMap.ALERT MapHeader.style.padding="1px 0px 0px 0px"; MapHeader.innerHTML="<img src='../images/Title_OiledWildlifeMap_White.png' alt='Map Title'>";
2. Changing the Credits
var Credits=TheCanvasMap.GetElement(CanvasMap.MAP_CREDITS); Credits.style.textAlign="right"; Credits.style.marginRight="5px"; Credits.innerHTML="Muhl, Kimble, Bean, and Graham<br>19th of August, 2015";
3. Changing the Displayed Spatial Refernce
var SRS=TheCanvasMap.GetElement(CanvasMap.MAP_SRS); SRS.innerHTML="Universal Transverse Mercator, Zone 10 North";
4. Changing the Coordinate Units
See the reference section for the CanvasMap class for the other coordinate unit types.
TheCanvasMap.SetCoordinateUnits(CanvasMap.COORDINATES_UNITS_METERS);
Change the Style of an Element in the CanvasMap
Since CanvasMap is made up of DOM elements, you can change the appearance of all the elements of your CanvasMap in a variety of ways:
1. New CSS file
If you want to change all the element's styles, you can save the CanvasMap.css file with a new name and/or new folder and point your HTML file to the new CSS file. Then, change the file as desired. If you look through the CSS file, you'll see that the styles are all named after the elements they represent in the map (see the first tutorial for an introduction to this and then the Reference section for details). Using this approach, you can change all the backgrounds, outlines, fonts, and even positioning of the elements.
2. Change one element
After calling Initialization() you can change the style of any element as follows:
var MapHeader=TheCanvasMap.GetElement(CanvasMap.MAP_HEADER); // obtains the element from your CanvasMap MapHeader.style.borderRadius="10px"; // changes the radius of the map header to 10 pixels
3. Dynamically change styles.
You can use the approach in item 2 above to change the styles whenever you desired. This could be when a tool or element is clicked on or with animation.
Replacing or Turning Off Elements
When you call "initialize()" CanvasMap creates the elements for the basic map. You can call the "SetElement()" function before Initialize() to replace the elements within CanvasMap with your own. This would allow you to create new DIV elements in other places on the page (or that float). You can also call "SetElement()" with "null" to "turn off" elements within CanvasMap.
TheCanvasMap.SetElement(CanvasMap.MAP_HEADER,null); // turn off the header with the map title
TheCanvasMap.SetElement(CanvasMap.TOOL_CONTAINER,null); // turn off the tool bar below the title
TheCanvasMap.SetElement(CanvasMap.NAVIGATION,null); // turn off the nagivation controls in the map
TheCanvasMap.SetElement(CanvasMap.TAB_CONTAINER,null); // turn off the tab controls to the upper right of the map
TheCanvasMap.SetElement(CanvasMap.LAYER_LIST,null); // hide the list of layers that is below the tab controls
TheCanvasMap.SetElement(CanvasMap.BACKGROUND_LIST,null); // hide the background list
TheCanvasMap.SetElement(CanvasMap.SEARCH_PANEL,null); // hide the search panel
TheCanvasMap.SetElement(CanvasMap.MAP_FOOTER,null); // hide the map footer at the bottom of the map
Adding Items on Top of the Map
There are slightly more advanced techniques that allow you to add your own content right on top of the map content.
Overriding a Scene's Paint Function
// save the existing function from the superclass TheScene.SuperClass_Paint=TheScene.Paint; // Override the existing mouse move with our own so we can chagne the action TheScene.MouseDown=function(TheView) { // call the superclass function this.Paint(TheView); // add any painting you want to have appear on top of the map here ... }
The map is drawn in "TheScene" object. You can override this objects "Paint()" function and then call the original function to have it draw as expected. Then you can add your own painting which will appear on top of the map. To be added:
Overlaying DIVs
Your map is drawn in an HTML 5 Canvas object. However, it is wrapped in a "CANVAS_CONTAINER" DIV element. This means you can add your own DIV elements to the CANVAS_CONTAINER to add elements on top of your map. This approach is used to create the legend in the census example.